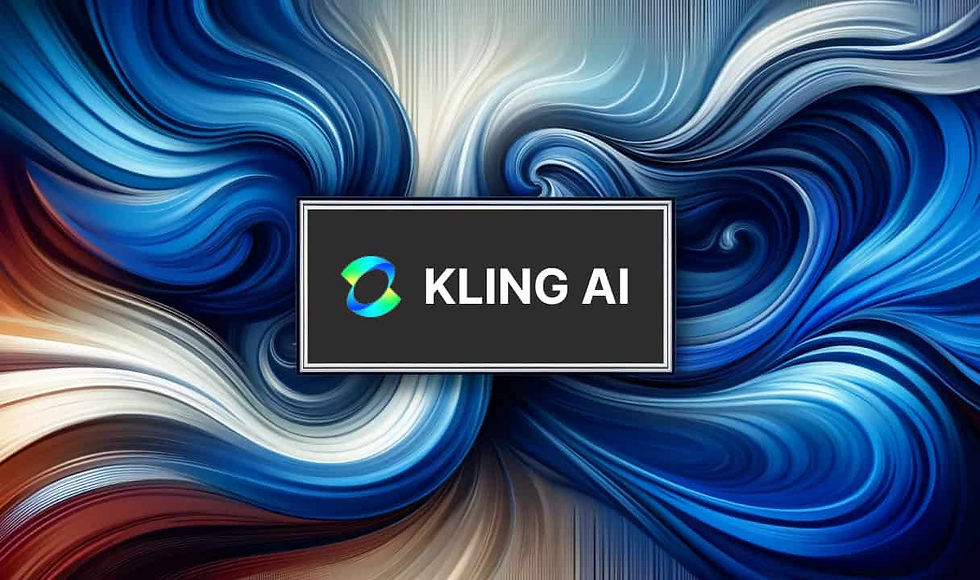
In this tutorial, we’ll walk through how to use the Kling AI API to generate images based on a text prompt. We’ll cover everything from setting up your environment to retrieving the generated images. Let’s get started!
The official documentation of Kling AI can be found here.
Prerequisites
Before we begin, make sure you have the following:
Python Installed: Ensure you have Python installed on your system. You can download it from python.org.
API Keys: You’ll need an API key (Access Key and Secret Key) from Kling AI. If you don’t have one, sign up on their platform to get your keys.
Required Libraries: Install the necessary Python libraries using pip:
pip install requests pyjwt
Step 1: Set Up Your Environment
First, create a new Python file (e.g., kling_image_generation.py) and import the necessary libraries:
import timeimport jwtimport requests
Step 2: Generate a JWT Token
Kling AI uses JWT (JSON Web Tokens) for authentication. You’ll need to generate a JWT token using your Access Key (AK) and Secret Key (SK).
Here’s a function to generate the JWT token:
def encode_jwt_token(ak, sk): headers = { "alg": "HS256", "typ": "JWT" } payload = { "iss": ak, # Your Access Key "exp": int(time.time()) + 1800, # Token expires in 30 minutes "nbf": int(time.time()) - 5 # Token is valid 5 seconds from now } return jwt.encode(payload, sk, headers=headers)
ak: Your Access Key.
sk: Your Secret Key.
exp: The expiration time of the token (30 minutes from now).
nbf: The “not before” time, which ensures the token is valid only after 5 seconds from now.
Step 3: Generate an Image
Now that you have a JWT token, you can use it to generate an image based on a text prompt.
Here’s a function to generate an image:
def generate_image(prompt, api_key, **kwargs): url = "https://api.klingai.com/v1/images/generations" headers = { "Content-Type": "application/json", "Authorization": f"Bearer {api_key}" } payload = {"model": "kling-v1", "prompt": prompt, **kwargs} return requests.post(url, headers=headers, json=payload).json()
prompt: The text description of the image you want to generate.
api_key: The JWT token generated in the previous step.
kwargs: Additional parameters you can pass to the API (e.g., width, height, etc.).
Step 4: Check the Task Status
After submitting the image generation request, you’ll receive a task ID. You’ll need to check the status of this task to retrieve the generated images.
Here’s a function to check the task status:
def get_task_result(task_id, api_key): url = "https://api.klingai.com/v1/images/generations" headers = {"Authorization": f"Bearer {api_key}"} params = {"pageSize": 500} response = requests.get(url, headers=headers, params=params).json() for task in response["data"]: if task["task_id"] == task_id and task["task_status"] == "succeed": return task["task_result"]["images"] return None
task_id: The ID of the task you want to check.
api_key: The JWT token.
Step 5: Wait for the Image to Be Generated
Image generation can take some time, so you’ll need to periodically check the task status until the image is ready.
Here’s a function to wait for the image:
def wait_for_image(task_id, api_key, max_attempts=30, delay=2): for _ in range(max_attempts): images = get_task_result(task_id, api_key) if images: return images time.sleep(delay) raise TimeoutError("Task took too long")
task_id: The ID of the task.
api_key: The JWT token.
max_attempts: The maximum number of times to check the task status.
delay: The delay (in seconds) between each check.
Step 6: Generate and Retrieve the Image
Now that everything is set up, you can generate an image and retrieve the image URLs.
Here’s how you can do it:
# Generate tokenauthorization = encode_jwt_token(ak, sk)# Define your promptprompt = 'An anime-style illustration of a joyful young boy running down a sunny, peaceful traditional Japanese village street with a cheerful white dog following him. The street is lined with charming wooden houses featuring tiled roofs, sliding doors, and small gardens filled with lush greenery and vibrant flowers. The art style is inspired by Studio Ghibli, with vibrant colors, soft lighting, and detailed textures that create a whimsical, nostalgic, and serene atmosphere. The scene is bright, with sunlight filtering through the trees, casting gentle shadows, and a sense of lively motion in the boy and the dog.'# Generate the imageresult = generate_image(prompt=prompt, api_key=authorization)print("API Response:", result)# Check if the image generation was successfulif result.get("code") == 0: images = wait_for_image(result["data"]["task_id"], authorization) print("Image URLs:", [img["url"] for img in images])
authorization: The JWT token.
prompt: The text description of the image.
result: The response from the API after generating the image.
images: The list of image URLs once the image is generated.
For the above prompt, here is the image generated by King:

Tips for Better Results
Be specific in your prompts
Include style details (e.g., “digital art”, “photorealistic”, “anime style”)
Mention lighting, colors, and composition in your prompt
Use clear and descriptive language
Conclusion
That’s it! You’ve successfully generated an image using the Kling AI API. You can now use this code as a starting point to create more complex applications or integrate image generation into your projects.
Remember to keep your API keys secure and never share them publicly. Happy coding!
Comments